Так как мою предыдущую
тему заблокировала уважаемая Антенна, то я решил создать новую. И снова здравствуйте, я пришел к вам опять со скриптом Sprite_Character.
Прошлую проблему мне решил Dead elf, я не хочу никого напрягать, но если кто сможет помочь, то пожалуйста.
Из-за этого скрипта большие чары (например ворот) отображаются не так как надо
а так.
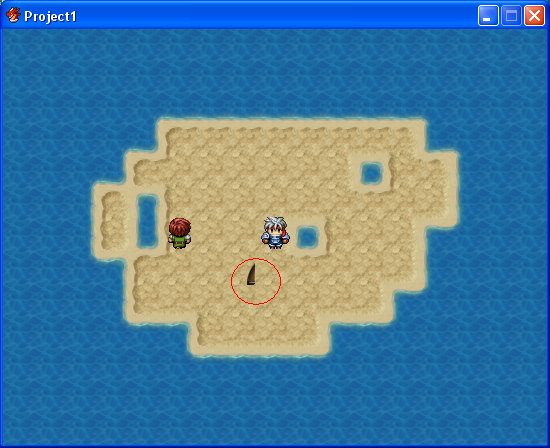
#==============================================================================
# ■ Sprite_Character
#------------------------------------------------------------------------------
# キャラクター表示用のスプライトです。Game_Character クラスのインスタンスを
# 監視し、スプライトの状態を自動的に変化させます。
#==============================================================================
class Sprite_Character < Sprite_Base
#--------------------------------------------------------------------------
# ● 公開インスタンス変数
#--------------------------------------------------------------------------
attr_accessor :character
#--------------------------------------------------------------------------
# ● オブジェクト初期化
# character : Game_Character
#--------------------------------------------------------------------------
def initialize(viewport, character = nil, battler = nil)
super(viewport)
@character = character
@balloon_duration = 0
@battler = battler
@battler_visible = false
@effect_type = nil
@effect_duration = 0
@damage_duration = 0
@charging_duration = 0
update
end
#--------------------------------------------------------------------------
# ● キャラクターネームを返す
#--------------------------------------------------------------------------
def character_name
name = @battler == nil ? @character.character_name : @battler.character_name
name
end
#--------------------------------------------------------------------------
# ● キャラクターインデックスを返す
#--------------------------------------------------------------------------
def character_index
index = @battler == nil ? @character.character_index : @battler.character_index
index
end
#--------------------------------------------------------------------------
# ● バトラーを設定
#--------------------------------------------------------------------------
def set_battler(battler)
@battler = battler
end
#--------------------------------------------------------------------------
# ● フレーム更新
#--------------------------------------------------------------------------
def update
super
update_bitmap
update_src_rect
update_position
update_other
update_balloon
setup_new_effect
if $game_temp.in_battle and @battler
setup_new_animation
update_effect
end
end
#--------------------------------------------------------------------------
# ● 転送元ビットマップの更新
#--------------------------------------------------------------------------
def update_bitmap
if graphic_changed?
@tile_id = @character.tile_id
@character_name = character_name
@character_index = character_index
if @tile_id > 0
set_tile_bitmap
else
set_character_bitmap
end
end
end
#--------------------------------------------------------------------------
# ● グラフィックの変更判定
#--------------------------------------------------------------------------
def graphic_changed?
@tile_id != @character.tile_id ||
@character_name != character_name ||
@character_index != character_index
end
#--------------------------------------------------------------------------
# ● キャラクターのビットマップを設定
#--------------------------------------------------------------------------
def set_character_bitmap
new_bitmap = Cache.character(@character_name)
if not $game_temp.in_battle
self.bitmap = new_bitmap
else
if bitmap != new_bitmap
self.bitmap = new_bitmap
init_visibility
end
end
sign = @character_name[/^[\!\$]/]
if sign && sign.include?('$')
@cw = bitmap.width / 3
@ch = bitmap.height / 4
else
@cw = bitmap.width / 12
@ch = bitmap.height / 8
end
self.ox = @cw / 2
self.oy = @ch
end
#--------------------------------------------------------------------------
# ● 可視状態の初期化
#--------------------------------------------------------------------------
def init_visibility
return if not @battler
@battler_visible = @battler.alive?
self.opacity = 0 unless @battler_visible
end
#--------------------------------------------------------------------------
# ● 転送元矩形の更新
#--------------------------------------------------------------------------
def update_src_rect
if @tile_id == 0
index = character_index
pattern = @character.pattern < 3 ? @character.pattern : 1
if not $game_temp.in_battle
sx = (index % 4 * 3 + pattern) * @cw
sy = (index / 4 * 4 + (@character.direction - 2) / 2) * @ch
else
sign = @character_name[/^[\!\$]./]
if sign && sign.include?('$')
sx = pattern * @cw
sy = index * @ch
else
sx = (index % 4 * 3 + pattern) * @cw
sy = (index / 4 * 4 + (@character.direction - 2) / 2) * @ch
end
end
self.src_rect.set(sx, sy, @cw, @ch)
end
end
#--------------------------------------------------------------------------
# ● その他の更新
#--------------------------------------------------------------------------
def update_other
self.opacity = @character.opacity if not $game_temp.in_battle
self.blend_type = @character.blend_type
self.bush_depth = @character.bush_depth
self.visible = !@character.transparent if not $game_temp.in_battle
end
#--------------------------------------------------------------------------
# ● 新しいエフェクトの設定
#--------------------------------------------------------------------------
def setup_new_effect
if !animation? && @character.animation_id > 0
animation = $data_animations[@character.animation_id]
start_animation(animation)
end
if !@balloon_sprite && @character.balloon_id > 0
@balloon_id = @character.balloon_id
start_balloon
end
return if not @battler
if !@battler_visible && @battler.alive?
start_effect(:appear)
elsif @battler_visible && @battler.hidden?
start_effect(:disappear)
end
if @battler.charging and @charging_duration == 0
start_effect(:whiten)
@charging_duration = 48
end
if @battler.damage_bar and @damage_duration == 0 and not @battler.boss_status_conceal?
@damage_duration = 36
@mini_hp_sprite = Sprite_Mini_Hp_Bar.new(viewport, @battler, @character)
@battler.damage_bar = false
end
if @battler_visible && @battler.sprite_effect_type
start_effect(@battler.sprite_effect_type)
@battler.sprite_effect_type = nil
end
end
#--------------------------------------------------------------------------
# ● エフェクトの開始
#--------------------------------------------------------------------------
def start_effect(effect_type)
@effect_type = effect_type
case @effect_type
when :appear
@effect_duration = 16
@battler_visible = true
when :disappear
@effect_duration = 32
@battler_visible = false
when :whiten
@effect_duration = 16
@battler_visible = true
when :blink
@effect_duration = 20
@battler_visible = true
when :collapse
@effect_duration = 48
@battler_visible = false
when :boss_collapse
@effect_duration = bitmap.height
@battler_visible = false
when :instant_collapse
@effect_duration = 16
@battler_visible = false
end
revert_to_normal
end
#--------------------------------------------------------------------------
# ● 通常の設定に戻す
#--------------------------------------------------------------------------
def revert_to_normal
self.blend_type = 0
self.color.set(0, 0, 0, 0)
self.opacity = 255
end
#--------------------------------------------------------------------------
# ● 新しいアニメーションの設定
#--------------------------------------------------------------------------
def setup_new_animation
if @battler.animation_id > 0
animation = $data_animations[@battler.animation_id]
mirror = @battler.animation_mirror
start_animation(animation, mirror)
@battler.animation_id = 0
end
end
#--------------------------------------------------------------------------
# ● エフェクト実行中判定
#--------------------------------------------------------------------------
def effect?
@effect_type != nil
end
#--------------------------------------------------------------------------
# ● 早送り判定
#--------------------------------------------------------------------------
def show_fast?
Input.press?(:A) || Input.press?(:C)
end
#--------------------------------------------------------------------------
# ● エフェクトの更新
#--------------------------------------------------------------------------
def update_effect
if @effect_duration > 0
@effect_duration -= 1
case @effect_type
when :whiten
update_whiten
when :blink
update_blink
when :appear
update_appear
when :disappear
update_disappear
when :collapse
update_collapse
when :boss_collapse
update_boss_collapse
when :instant_collapse
update_instant_collapse
end
@effect_type = nil if @effect_duration == 0
end
if @charging_duration > 0
update_charging
end
if @damage_duration > 0
@damage_duration -= show_fast? ? 2 : 1
@mini_hp_sprite.show_fast(show_fast?)
@mini_hp_sprite.update
if @damage_duration <= 0
@mini_hp_sprite.dispose
@damage_duration = 0
end
end
end
#--------------------------------------------------------------------------
# ● 白フラッシュエフェクトの更新
#--------------------------------------------------------------------------
def update_whiten
self.color.set(255, 255, 255, 0)
self.color.alpha = 128 - (16 - @effect_duration) * 10
end
#--------------------------------------------------------------------------
# ● 点滅エフェクトの更新
#--------------------------------------------------------------------------
def update_blink
self.opacity = (@effect_duration % 10 < 5) ? 255 : 0
end
#--------------------------------------------------------------------------
# ● 出現エフェクトの更新
#--------------------------------------------------------------------------
def update_appear
self.opacity = (16 - @effect_duration) * 16
end
#--------------------------------------------------------------------------
# ● 消滅エフェクトの更新
#--------------------------------------------------------------------------
def update_disappear
self.opacity = 256 - (32 - @effect_duration) * 10
end
#--------------------------------------------------------------------------
# ● 崩壊エフェクトの更新
#--------------------------------------------------------------------------
def update_collapse
self.blend_type = 1
self.color.set(255, 128, 128, 128)
self.opacity = 256 - (48 - @effect_duration) * 6
end
#--------------------------------------------------------------------------
# ● ボス崩壊エフェクトの更新
#--------------------------------------------------------------------------
def update_boss_collapse
alpha = @effect_duration * 120 / bitmap.height
self.x = @character.screen_x + @effect_duration % 2 * 4 - 2
self.y += bitmap.height / 8 - @effect_duration / 8
self.blend_type = 1
self.color.set(255, 255, 255, 255 - alpha)
self.opacity = alpha
Sound.play_boss_collapse2 if @effect_duration % 20 == 19
end
#--------------------------------------------------------------------------
# ● 瞬間崩壊エフェクトの更新
#--------------------------------------------------------------------------
def update_instant_collapse
self.opacity = 0
end
#--------------------------------------------------------------------------
# ● 詠唱中アニメの更新
#--------------------------------------------------------------------------
def update_charging
@charging_duration -= 1
end
end